A lengthy application loading time is one of the most common user experience issues that developers encounter. In this article, you will learn how optimizing web assets can significantly speed up load times. We will explore various methods for testing web performance and provide practical strategies for improving key performance metrics.
Let’s commence by clarifying the concept of “web assets.” In the context of web applications, the term “assets” refers to the various types of resources or files used to display content and features. Here are examples of typical assets on websites:
- Images: pictures, graphics, illustrations, icons, backgrounds and other visual elements
- Multimedia: video and audio files, animations
- Fonts: Fonts or font files that are used to determine the appearance of text
- Source codes (HTML, CSS, JavaScript): they are also considered assets because they are necessary for creating and rendering web pages. HTML defines the structure of the page, CSS is responsible for styling, and JavaScript is used for interactivity.
Table of Contents
Fundamentals of User Experience
User Experience (UX) in the context of software refers to the overall experience of a user while using it. The goal of good UX is to ensure that user interactions are intuitive and effective. These are the key elements of UX in the context of web applications:
- Usability – The application should be easy to use, allowing users to perform desired actions easily and quickly.
- Accessibility – The application should be accessible to people with various limitations, which includes adapting the interface to the needs of people with disabilities.
- Response time – The application’s response time to user interactions is crucial. Loading delays can negatively impact the user experience and discourage further use of the app.
- Interactivity – The interaction design should encourage engagement, be logical, and ensure a smooth experience, which will increase the overall satisfaction of using the application.
Effective UX practice in web applications involves ongoing testing and optimization of these elements to provide users with an enjoyable and productive experience, which in turn can lead to increased user satisfaction. In this article, we will focus on response time as a critical aspect of web performance.
Fundamental metrics for assessing web performance in the context of Core Web Vitals
Largest Contentful Paint
Largest Contentful Paint (LCP) is one of the most significant metrics in Core Web Vitals, measuring the time it takes to load the largest visual element on a website. According to Google’s definition, this metric only considers the visible page content above the fold, meaning the content that initially appears without scrolling, and should ideally take up to 2.5 seconds to load.
First Contentful Paint
First Contentful Paint (FCP) is another crucial metric that measures the time it takes for the browser to render the first DOM element containing content. This metric is important for ensuring that visible elements load quickly. To provide the best user experience, websites should aim for an FCP of 1.8 seconds or less.
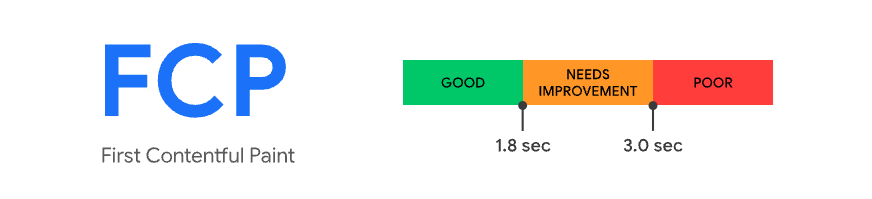
Cumulative Layout Shift
Cumulative Layout Shift (CLS) measures visual stability by assessing how much the page layout shifts during loading. It is crucial for ensuring the stability of the page layout. The score should be 0.1 or lower.
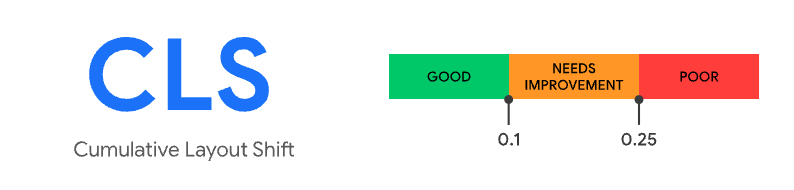
Optimizing assets such as images, fonts, and multimedia is crucial for reducing metric times. When assets are well optimized, they become easier to download and render, thereby enhancing app performance and improving these metrics.
How to optimize images and multimedia
Image compression
In the context of website optimization, image compression involves reducing the size of image files to make the page load faster. There are several image compression methods:
- Lossy compression involves removing some information from the image and reducing its resolution, resulting in a file with a significantly reduced size. Examples of lossy compression formats include JPEG.
- Lossless compression reduces the file size without losing image quality. Examples of lossless compression formats include PNG and GIF. However, compared to lossy compression, lossless compression typically results in smaller size reductions.
- Format WebP: This format supports both lossy and lossless compression, enabling significant file size reduction with minimal quality loss. Nearly all modern web browsers now support this format, making it a versatile choice for programmers.
- AVIF (AV1 image file format): This format offers excellent compression rates and is intended to replace both JPEG and WebP formats. Similar to the WebP format, it supports both lossy and lossless compression and is compatible with most modern web browsers.
- JPEG XL: This is a newer image format intended to replace JPEG, providing better compression performance and support for features such as progressive rendering and animation.
- HEIF (High-Efficiency Image File Format): Although the HEIF format is more commonly used in Apple devices, it can contain images and image sequences compressed using HEVC (High Efficiency Video Coding). This format provides better compression than JPEG and supports features like transparency, 16-bit color, and multiple images in one file, which is perfect for burst photos and image sequences.
- Optimizing images for the web: It’s worth adjusting the size and quality of images to the application’s actual requirements. We don’t always need the highest-quality image. Image optimization tools such as ImageOptim, Squoosh, and TinyPNG automatically reduce file sizes while maintaining acceptable quality.
Below is a comparison of the same image in JPG, WebP, and AVIF formats. This example demonstrates how the WebP and AVIF formats significantly reduce file size without a visible loss in image quality. The conversion to WebP was performed using a simple one-line script: cwebp input.jpg -o output.webp
. The conversion to AVIF was similarly done using a one-liner from libavif
: avifenc input.jpg output.avif
.
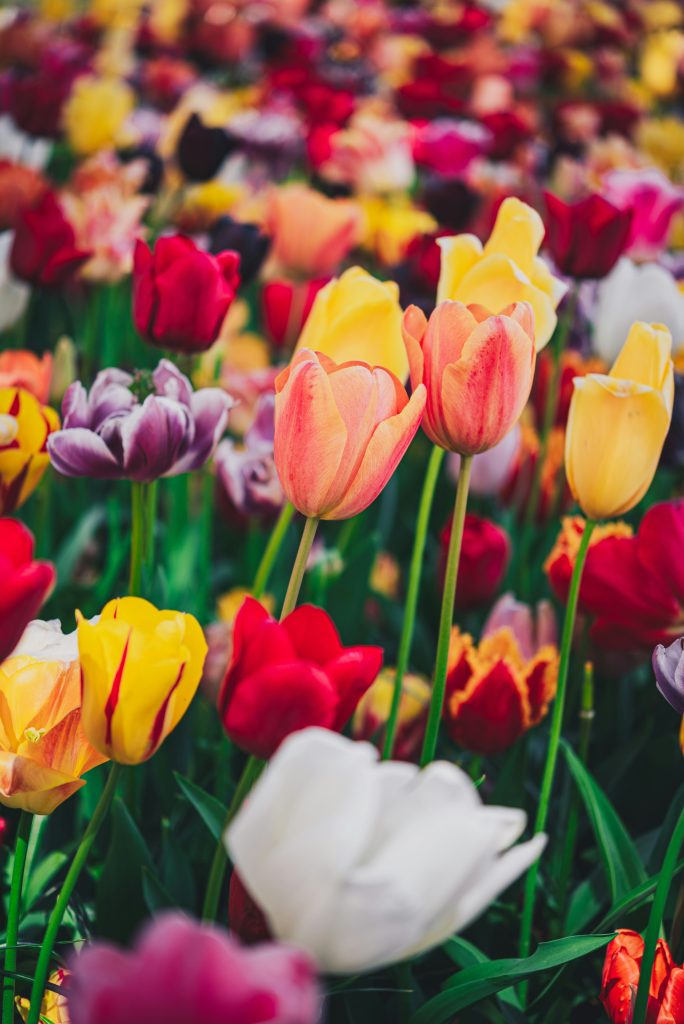
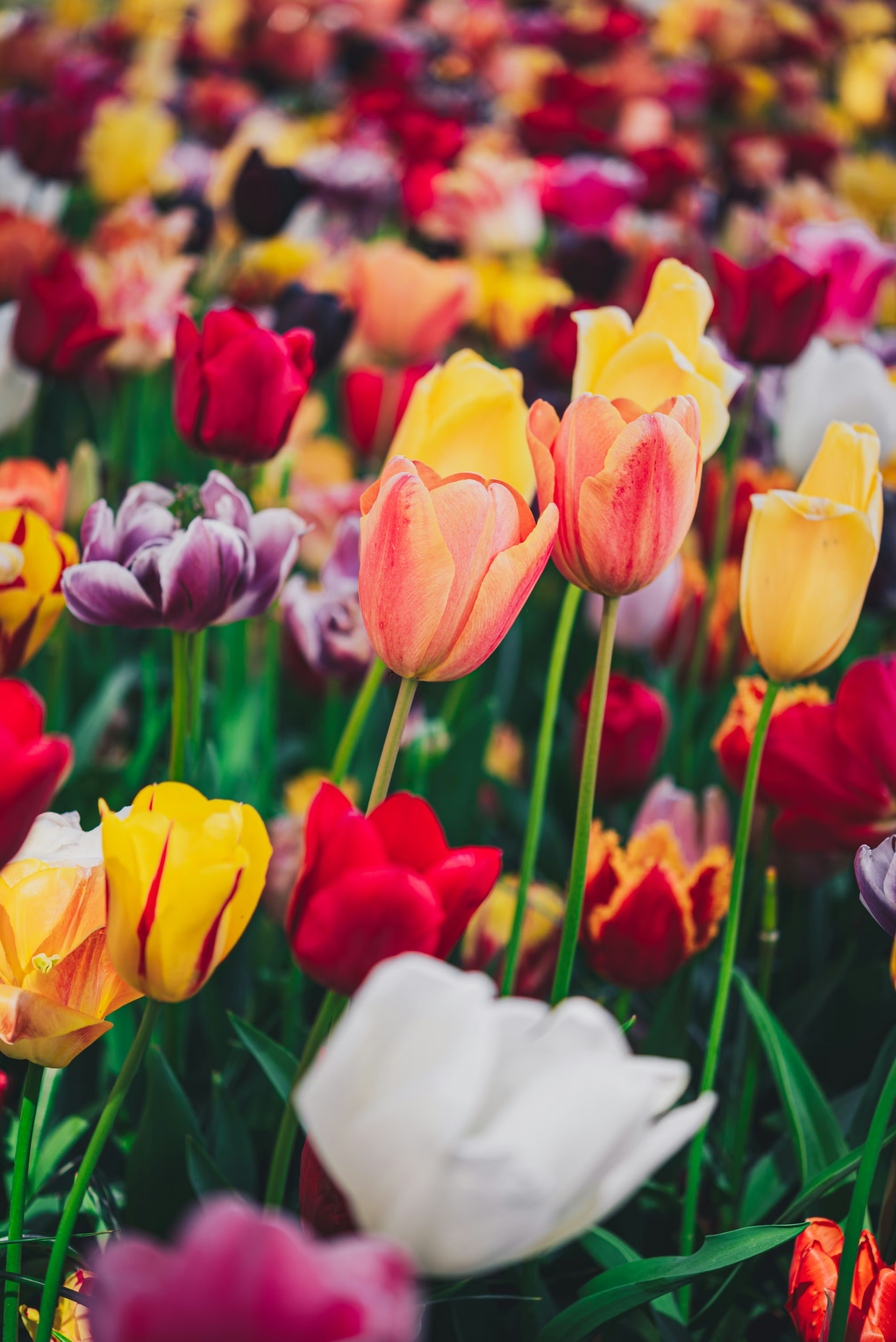
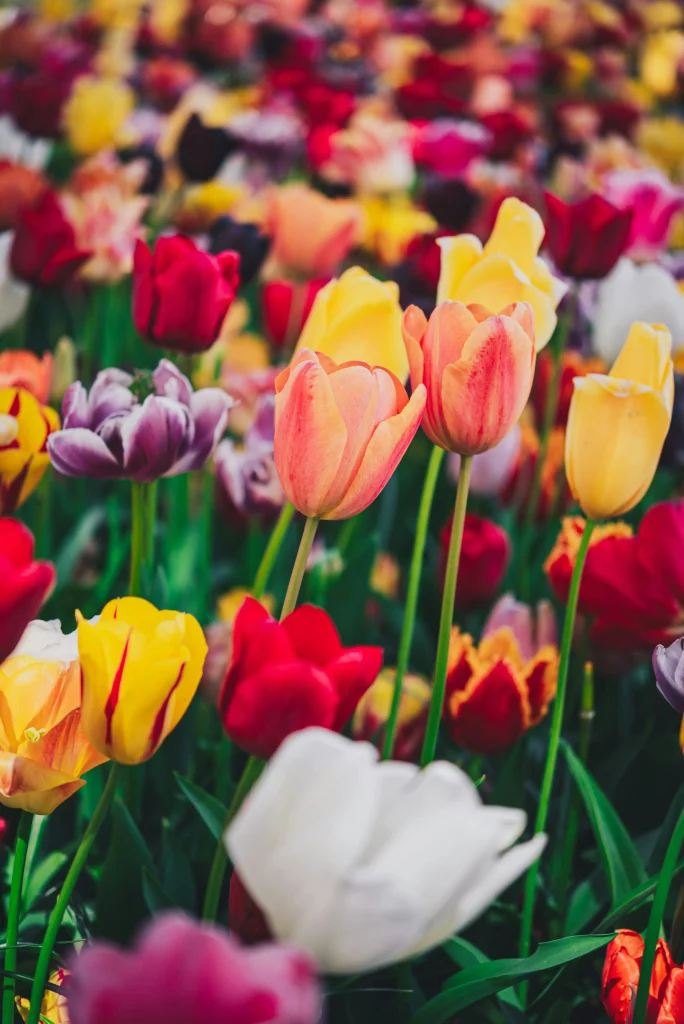
Lazy Loading
Implementing lazy loading allows images to be loaded only when they are visible on the user’s screen. This helps minimize the amount of data that needs to be downloaded at the beginning of the page load.
Lazy loading for image:
<img src="lazy-loaded-image.jpg" alt="Image description" loading="lazy">
In this case, loading="lazy"
is used as an attribute in the image tag. The image will be loaded as soon as it appears in the user’s visibility area.
Lazy loading for iframe:
<iframe src="lazy-loaded-video.html" width="640" height="360" frameborder="0" allowfullscreen loading="lazy"></iframe>
In the case of iframes, we can also use loading="lazy"
. This will cause an iframe (e.g., with an embedded video) to be loaded when it appears in the user’s viewport.
Use BlurHash for image placeholders
When discussing image optimization techniques like lazy loading, a tool like BlurHash is also worth mentioning. Lazy loading is an effective way to delay the loading of images until they are needed, which helps to load the page faster. However, lazy loading alone does not solve the problem of empty space before the image is loaded. In this context, BlurHash can be a valuable addition because it allows you to display an aesthetically pleasing blurred outline of an image before it is completely loaded.
Adaptive image variants
Adaptive image variants involve using different image versions tailored to various screen sizes (responsive images), allowing you to deliver optimal images to users on different devices.
To implement adaptive image variations in an application, the <picture>
element can be utilized in combination with the srcset
and sizes
attributes to serve different image resolutions based on the screen size of the device. Here’s an example:
<picture>
<!-- Image variant for large screens (above 768px) -->
<source media="(min-width: 768px)" srcset="large-image.jpg" sizes="(max-width: 1024px) 50vw, 800px">
<!-- Image variant for medium screens (above 480px) -->
<source media="(min-width: 480px)" srcset="medium-image.jpg" sizes="(max-width: 767px) 70vw, 480px">
<!-- Default image variant for small screens -->
<img src="small-image.jpg" alt="Image description">
</picture>
In this setup:
- The first
<source>
tag specifies an image for screens wider than 768px. The ‘sizes’ attribute indicates that on screens up to 1024px wide, the image should take up 50% of the viewport width but should be 800px wide when the viewport is larger.
- The second
<source>
tag caters to medium screens with a width of 480px to 768px. Here, the image will take up 70% of the viewport width on screens narrower than 768px but will be 480px wide on larger screens.
- The
<img>
tag provides a default image for smaller screens and devices where none of the conditions in the<source>
tags are met.
This approach enhances responsive web design by adapting the image size dynamically according to the screen size, improving both the visual quality and the load times of your app. This method is particularly beneficial for designs that need to accommodate a wide range of devices and screen resolutions.
CDN (Content Delivery Network)
Utilize CDN services to deliver assets from servers that are closer to the users. This can reduce the access time to assets and enhance performance. By leveraging a distributed network of servers strategically located around the globe, CDNs store copies of your web and application assets (such as images, videos, CSS, and JavaScript files) closer to where your users are. This geographical proximity significantly decreases the amount of time it takes for your content to travel from the server to the user’s device, leading to faster page load times and improved user experience. Additionally, CDNs can offer added benefits like load balancing, which helps in managing traffic spikes, and protection against DDoS attacks, ensuring your web and application infrastructure remains robust and reliable.
Use only a bearable minimum of fonts.
When selecting fonts, aim to limit yourself to one or two font families. Using more can significantly increase page load times. Consider using system fonts, which are pre-installed on most devices and do not require additional downloads. Here are examples of how to use system fonts in your CSS:
For Apple devices (macOS and iOS):
body {
font-family: -apple-system, BlinkMacSystemFont, 'Segoe UI', 'Roboto', 'Helvetica Neue', Arial, sans-serif;
}
For Windows devices:
body {
font-family: "Segoe UI", Tahoma, Geneva, Verdana, sans-serif;
}
For general serif fonts across different systems:
body { font-family: ui-serif, Georgia, Cambria, "Times New Roman", Times, serif; }
Each font family can have multiple weights (e.g., bold, light, regular) and styles (e.g., italic). To reduce file downloads, limit them to the essential ones, like regular and one bold version. For instance, instead of loading font weights 100, 200, 300, 400, 500, and 600 separately, just use 400 and 500.
Make sure the fonts are compressed (e.g., WOFF2 format, which is more efficient than TTF or OTF).
Use font-display: swap
in CSS to display text in a fallback font until the main font loads.
It’s important to include the necessary @font-face
or @import
rules in your CSS to ensure that custom fonts are properly loaded by the browser. Here’s an example from Tailwind CSS showing how to include a custom font:
@font-face {
font-family: 'Roboto';
font-style: normal;
font-weight: 400;
font-display: swap;
src: url(/fonts/Roboto.woff2) format('woff2');
}
CSS code optimization
CSS code compression and minification are basic optimization techniques. A tool like CSSNano can remove unnecessary characters, whitespace, and comments, reducing file sizes and speeding up page loading.
Libraries and plugins can significantly increase page size and loading time. Use them sparingly and only when you really need them. Consider alternatives such as native browser features or lighter libraries that offer similar functionality.
Review and remove unused CSS code regularly. Tools like PurifyCSS, Lightning CSS, and UnCSS can automatically detect and remove unused code, helping to reduce file sizes.
JavaScript code optimization
JavaScript code optimization is similarly extremely important for improving the performance and speed of web applications.
Just like CSS, JavaScript code also needs to be minified. Tools such as Terser or UglifyJS can be used to minify JavaScript code, reducing its size and improving load times.
In addition to minimizing and combining scripts, we should consider using modern JavaScript frameworks and libraries that are designed to increase web performance. For example, HTMX allows us to use AJAX, CSS Transitions, and WebSockets directly in HTML, greatly simplifying the application development process. Similarly, the Astro framework allows the building of faster websites by providing less JavaScript and using partial hydration of components only when necessary.
The use of async
and defer
attributes for JavaScript scripts allows for simultaneous loading of resources without blocking page rendering. async
loads scripts simultaneously with other resources, and defer
delays their execution until the page is fully loaded.
Use bundling (combining multiple files into one) and tree shaking (removing unused parts of the code) techniques in the application-building process. Tools like Webpack and Rollup can automatically optimize your code, improving its performance.
These tools and practices help optimize the code base, reduce load times, and improve the overall user experience.
Third-party scripts optimization
Optimizing third-party scripts is equally valid for enhancing website performance. These scripts, often coming from third-party services such as live chats, ads, analytics, and social media buttons, can significantly impact page loading speeds. One tool that allows the optimization of these scripts is Partytown.
Partytown is a library that allows third-party script execution to be off-loaded to Web Workers, isolating it from the main browser thread. This tool allows scripts to be executed without affecting the performance of interactive page elements, resulting in faster load times and enhanced user experiences.
In general, it is worth considering what third-party scripts are actually needed and regularly assessing their impact on the app’s performance. Ideally, reduce the number of these scripts to the minimum or find lighter alternatives that offer similar functionality without adding high weight to the site.
Test your application’s performance using free tools
WebPageTest is a popular website performance analysis tool that enables detailed analysis of asset loading.
Features: Loading speed tests, first view analysis, image optimization, video loading analysis.
Advantages: Detailed waterfall reports and the ability to test from different locations and browsers.
Google PageSpeed Insights evaluates app performance on desktops and mobile devices using various metrics and offers suggestions for resource optimization.
Features: Performance analysis and recommendations for optimizing images, scripts, and styles.
Advantages: Ease of use, direct guidance from Google.
Lighthouse is Google’s automated inspecting tool. It offers detailed reports on various performance aspects, including asset optimization.
Features: Performance, accessibility, Progressive Web Apps, Core Web Vitals reports.
Advantages: Versatility, integration with Chrome DevTools, detailed reports.
GTmetrix analyzes website performance, focusing on asset optimization and offering detailed recommendations.
Features: Loading speed tests, web assets analysis, optimization suggestions.
Advantages: Detailed waterfall reports, ability to compare results and historical data.
Examples of performance tests for famous web applications
In order to demonstrate the effectiveness of website performance testing tools, we will conduct tests for two well-known web applications: GitHub and Wikipedia.
GitHub testing
Goal: Evaluate GitHub page performance using PageSpeed Insights and Lighthouse.
Test using PageSpeed Insights
Steps:
- Open PageSpeed Insights.
- Enter the URL: https://www.github.com/.
- Do your analysis.
Results:
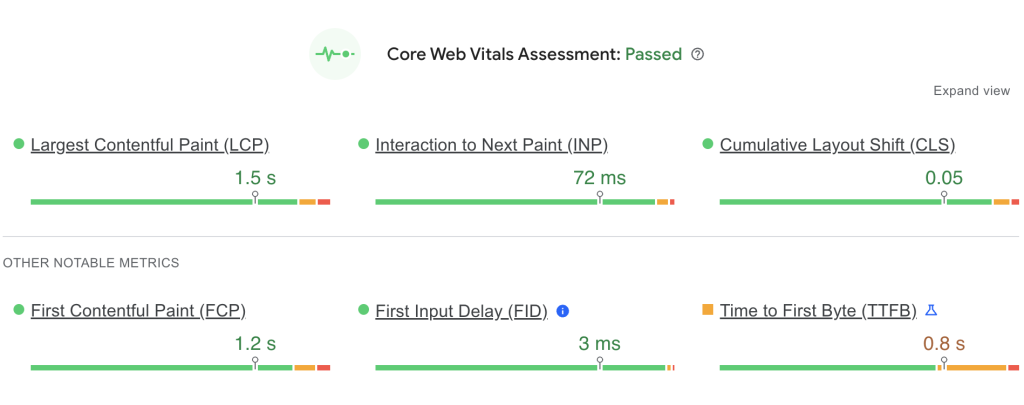
Test using Lighthouse
Steps:
- Install the Lighthouse Web Vitals extension in your Chrome DevTools.
- Enter the URL: https://www.github.com/.
- Do your analysis.
Results:
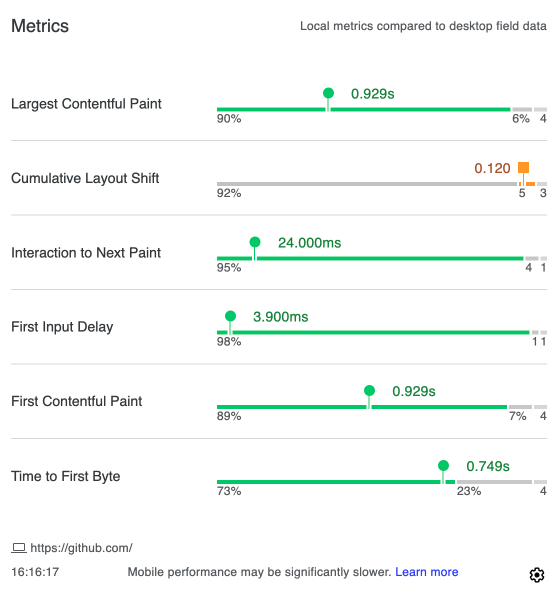
Summary of PageSpeed Insights and Lighthouse test results for GitHub:
PageSpeed Insights: The test was passed, but a longer time to the first byte (TTFB) of 0.8 seconds was observed, which is slightly above the recommended norm.
Lighthouse: Cumulative Layout Shift (CLS) was 1.2 seconds, which is also above the recommended values.
The remaining metrics in both tests are within normal limits, indicating good overall application performance.
Wikipedia testing
Goal: Evaluate Wikipedia page performance using PageSpeed Insights and Lighthouse.
Test using PageSpeed Insights
Steps:
- Open PageSpeed Insights.
- Enter the URL: https://www.wikipedia.org/.
- Do your analysis.
Results:
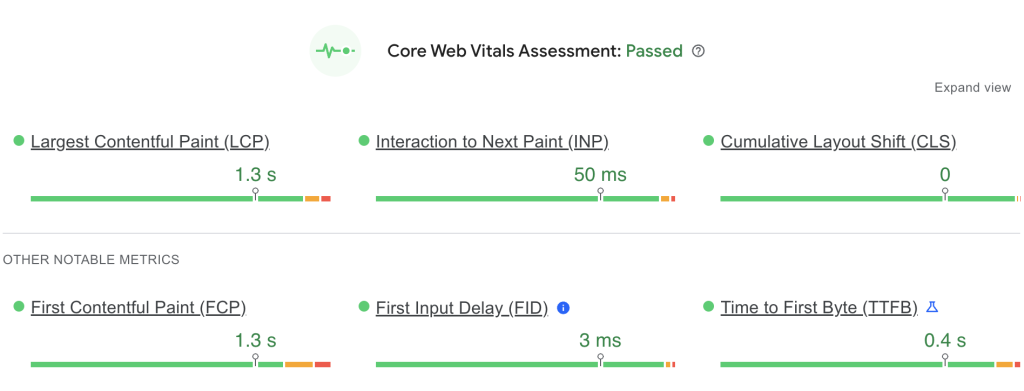
Test using Lighthouse
Steps:
- Install the Lighthouse Web Vitals extension in your Chrome DevTools.
- Enter the URL: https://www.wikipedia.org/.
- Do your analysis.
Results:
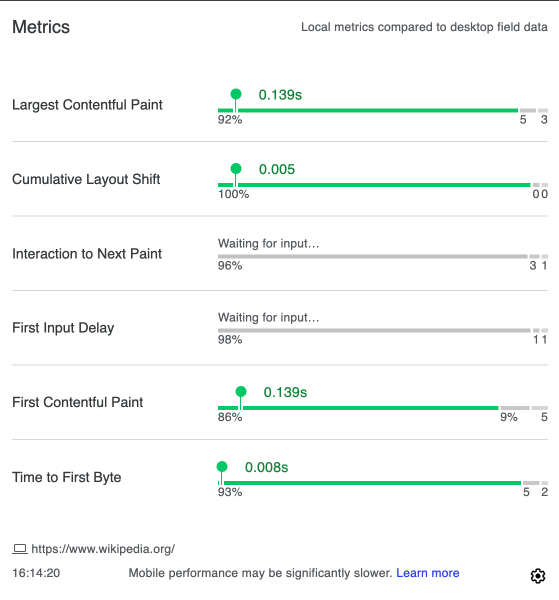
Summary of PageSpeed Insights and Lighthouse test results for Wikipedia:
PageSpeed Insights: All metrics are within the recommended norm, indicating excellent performance.
Lighthouse: All metrics meet the recommended values, demonstrating optimal performance.
Overall, the website shows outstanding performance across both tests.
Summary
Optimizing web assets is crucial for ensuring the high performance of web applications. Managing resources such as images, fonts, CSS, and JavaScript has a direct impact on page loading speeds, which translates into better user experiences and higher rankings in search results.
In particular, optimizing images through compression and lazy loading, minimizing CSS and JavaScript code, and limiting the number and variety of fonts are key steps in improving website performance. Regular application testing using tools such as PageSpeed Insights and Lighthouse allows you to identify areas requiring optimization and implement effective improvements.
Remember that continuous monitoring and optimization of web assets should be an integral part of the development of any web application to ensure its speed, reliability, and better user experience.
Making your platform faster makes you money quicker.
Wanna chat?Aneta Narwojsz is a dedicated Frontend Developer at Makimo, harnessing her profound expertise in JavaScript and other frontend technologies to deliver remarkable web solutions. Known for her knack for translating complex JavaScript fundamentals into easily digestible content, she regularly publishes enlightening articles and engaging video materials. When she's not immersed in code, Aneta unwinds with her favorite pastimes: visiting the cinema, immersing herself in books, experimenting in the kitchen, and exploring fashion trends.